I share example how to integration OAuth2 - Spring Boot2 - Security - JDBC Authentication.
Technologies used:
1. Spring Boot 2.0.3.RELEASE
2. Maven 4
3. Java 8
4. OAuth2
5. Eclipse: Oxygen.1.a Release (4.7.1a)
6. Spring Tools Suite (STS) 4
7. MySql
Code on GitHub:
https://github.com/HenryXiloj/SSO-OAuth2-SpringBoot2-JDBC
Steps:
Step 1:
Create tables on mysql:
CREATE TABLE users
(
username VARCHAR(50) NOT NULL PRIMARY KEY,
password VARCHAR(100) NOT NULL,
enabled BOOLEAN NOT NULL
);
CREATE TABLE authorities
(
username VARCHAR(50) NOT NULL,
authority VARCHAR(50) NOT NULL,
CONSTRAINT fk_authorities_users FOREIGN KEY(username) REFERENCES users(
username)
);
CREATE UNIQUE INDEX ix_auth_username ON authorities (username, authority);
Step 2: Insert your user, example:
For insert password on Databases you need to passwordEncoder, for example use Test.java for generate passwordEncoder:
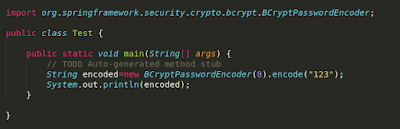
Resul is : $2a$08$sqfU5.UM.oEqgzVniREsvOsQ9eDtSD/xX8RXT2Bc4AmXWHo4fcchG
INSERT INTO users
VALUES ('henry',
'$2a$08$ykZFaf/pmTxnShaj5dqXZeWXiK3JJSJr5f20hqiVGSO35KNyQ0lgO'
/*123*/,
true);
INSERT INTO authorities
(username,
authority)
VALUES ('henry',
'ROLE_ADMIN');
Step 3:
On the proyect spring-security-sso-auth-server change the configuration in application.yml
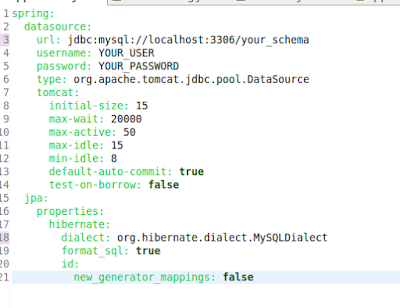
Step 4:
Run 2 proyects on Eclipse or if you prefer use command line on console:
First: auth server
Righ click on proyect -> Run As -> Spring Boot App
Expose on port: 8081
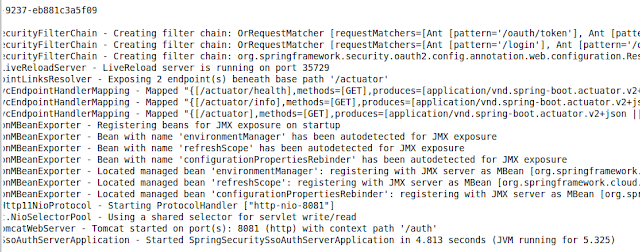
Second: sso-ui
Expose on port: 8082
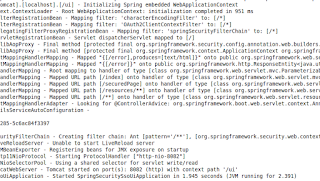
Step 5:
http://localhost:8082/ui/
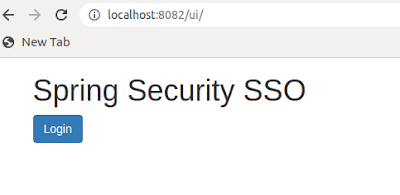
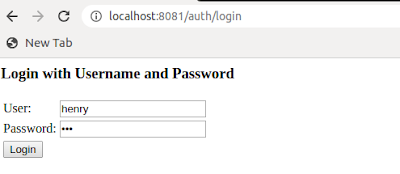
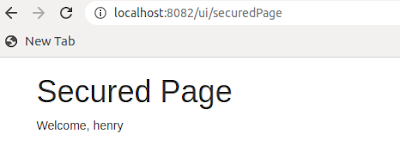
References:
https://www.baeldung.com/sso-spring-security-oauth2
https://www.baeldung.com/spring-security-jdbc-authentication
https://mkyong.com/spring-security/spring-security-form-login-using-database/
https://github.com/HenryXiloj/SSO-OAuth2-SpringBoot2-JDBC
Steps:
Step 1:
Create tables on mysql:
CREATE TABLE users
(
username VARCHAR(50) NOT NULL PRIMARY KEY,
password VARCHAR(100) NOT NULL,
enabled BOOLEAN NOT NULL
);
CREATE TABLE authorities
(
username VARCHAR(50) NOT NULL,
authority VARCHAR(50) NOT NULL,
CONSTRAINT fk_authorities_users FOREIGN KEY(username) REFERENCES users(
username)
);
CREATE UNIQUE INDEX ix_auth_username ON authorities (username, authority);
Step 2: Insert your user, example:
For insert password on Databases you need to passwordEncoder, for example use Test.java for generate passwordEncoder:
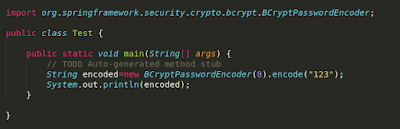
Resul is : $2a$08$sqfU5.UM.oEqgzVniREsvOsQ9eDtSD/xX8RXT2Bc4AmXWHo4fcchG
INSERT INTO users
VALUES ('henry',
'$2a$08$ykZFaf/pmTxnShaj5dqXZeWXiK3JJSJr5f20hqiVGSO35KNyQ0lgO'
/*123*/,
true);
INSERT INTO authorities
(username,
authority)
VALUES ('henry',
'ROLE_ADMIN');
Step 3:
On the proyect spring-security-sso-auth-server change the configuration in application.yml
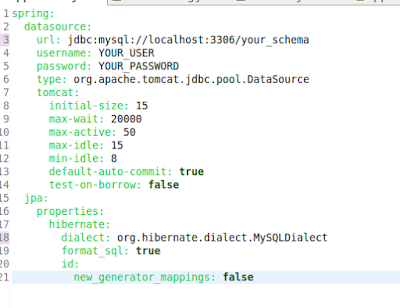
Step 4:
Run 2 proyects on Eclipse or if you prefer use command line on console:
First: auth server
Righ click on proyect -> Run As -> Spring Boot App
Expose on port: 8081
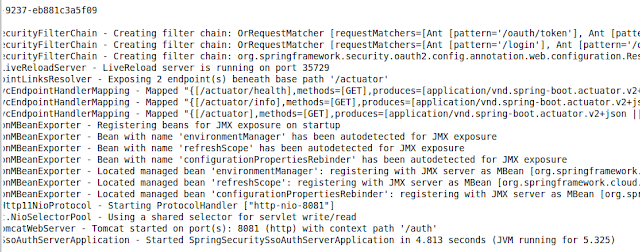
Second: sso-ui
Expose on port: 8082
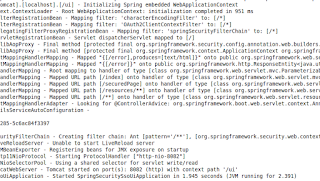
Step 5:
http://localhost:8082/ui/
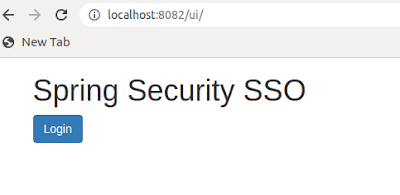
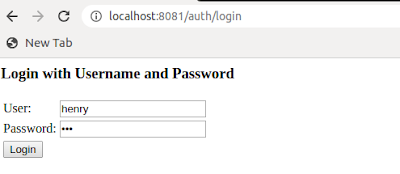
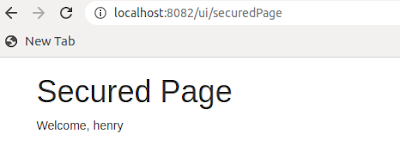
References:
https://www.baeldung.com/sso-spring-security-oauth2
https://www.baeldung.com/spring-security-jdbc-authentication
https://mkyong.com/spring-security/spring-security-form-login-using-database/