I share example how java Transformer outputs < and > instead of <> on XML.
/**
Example
*/
import java.io.StringReader;
import java.io.StringWriter;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.transform.OutputKeys;
import javax.xml.transform.Transformer;
import javax.xml.transform.TransformerFactory;
import javax.xml.transform.dom.DOMSource;
import javax.xml.transform.stream.StreamResult;
import org.apache.commons.lang.StringEscapeUtils;
import org.w3c.dom.Document;
import org.xml.sax.InputSource;
public class Test {
public static void main(String[] args) throws Exception {
// TODO Auto-generated method stub
String xml = "<?xml version=\"1.0\" encoding=\"UTF-8\" standalone=\"yes\"?>" +
"<company>" +
"<staff id=\"1001\">" +
"<firstname>henry</firstname>" +
"</staff>" +
"</company>";
DocumentBuilderFactory dbFactory = DocumentBuilderFactory.newInstance();
DocumentBuilder dBuilder = dbFactory.newDocumentBuilder();
Document doc = dBuilder.parse(new InputSource(new StringReader(StringEscapeUtils.unescapeXml(xml.toString()))));
System.out.println("final xml " + xmlTransformerInput(doc).toString());
}
public static String xmlTransformerInput(Document fDoc) {
try {
fDoc.setXmlStandalone(true);
DOMSource docSource = new DOMSource(fDoc);
Transformer transformer = TransformerFactory.newInstance().newTransformer();
transformer.setOutputProperty(OutputKeys.METHOD, "xml");
transformer.setOutputProperty(OutputKeys.ENCODING, "UTF-8");
transformer.setOutputProperty(OutputKeys.INDENT, "yes");
StringWriter sw = new StringWriter();
transformer.transform(docSource, new StreamResult(sw));
return sw.toString();
} catch (Exception e) {
e.printStackTrace();
return null;
}
}
}
Subscribe to:
Post Comments (Atom)
Integrating Google Cloud Pub/Sub with Terraform and Spring Boot 3 (Java 21)
Introduction In this blog post, I'll demonstrate how to provision Google Cloud Pub/Sub resources using Terraform and integrate them with...
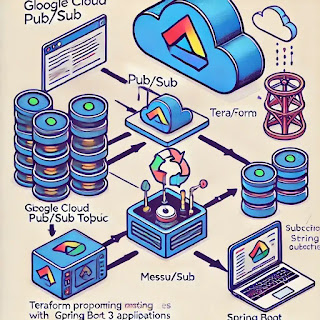
-
SAML V2.0 SAML version 2.0 was approved as an OASIS Standard in March 2005. Approved Errata for SAML V2.0 was last produced by the SSTC on 1...
-
Spring Boot 3 Spring boot 3 Features : Spring Boot 3.0 will require Java 17 or later Jakarta EE 9 a new top-level jakarta package, replacin...
-
In modern applications, transient failures (e.g., network timeouts, database connection issues, or external API unavailability) are inevitab...
No comments:
Post a Comment